RSS (Really Simple Syndication) was designed as a syndication format for website. There are some great websites that every individual likes to vist on a hourly/daily/weekly basis. How good would if be if we subscribe to the site and we receive emails from the4 site. That’s great. But then slowly that becomes a nagging point as we may have to open emails to know where we wish to go. Instead it will be good if we take the intermediate human readable form and have it read by computer programs. To get this done we need a data representation that is platform neutral – XML (Any argument)
RSS – XML format
Here are the rules for RSS XML format
- Top most element for RSS is <rss>
- Followed by <channel>. Channel describes the site syndicating the content and the content itself
- <channel> contains many element, but the most important one’s are <title><link>, <description>
- <item> element is used to provide information about a single syndicated data.
A snapshot of rss content from www.msdn.com/rss.xml
<rss version="2.0">
<channel>
<title>MSDN: U.S. Local Highlights</title>
<description>The latest developer information for the United States.</description>
<link>http://msdn.microsoft.com</link>
<language>en-us</language>
<lastBuildDate>Sun, 30 Aug 2009 06:10:00 GMT</lastBuildDate>
<pubDate>Sun, 30 Aug 2009 06:10:00 GMT</pubDate>
<ttl>1440</ttl>
<generator>FeedForAll v2.0 (2.0.2.9) http://www.feedforall.com</generator>
<item>
<title>Jumpstart Your Product Planning at PDC09 This November</title>
<description>Check out our sessions list to get a view into products, technologies, and developer tools that will shape the future of your business. Register by September 15 to save US$500 on your conference pass.</description>
<link>http://go.microsoft.com/?linkid=9681422</link>
<mscom:simpleDate>Aug 30</mscom:simpleDate>
<pubDate>Sun, 30 Aug 2009 06:10:00 GMT</pubDate>
</item>
<item>
<title>Windows 7 Direct Access</title>
<description>Watch this webcast to see how Direct Access in Windows 7 provides secure access to the network from any location, making management easier and reducing IT costs.</description>
<link>http://go.microsoft.com/?linkid=9681423</link>
<mscom:simpleDate>Aug 30</mscom:simpleDate>
<pubDate>Sun, 30 Aug 2009 06:10:00 GMT</pubDate>
</item>
<item>
<title>WPF Control Verifier Tool Version 0.1 Released</title>
<description>WPF Control Verifier is a tool that verifies the correctness of WPF controls. This tool is geared towards WPF control developers with the goal of providing a set of verifications that all controls can run and consume.</description>
<link>http://go.microsoft.com/?linkid=9681424</link>
<mscom:simpleDate>Aug 30</mscom:simpleDate>
<pubDate>Sun, 30 Aug 2009 06:10:00 GMT</pubDate>
</item>
</channel>
</rss>
Consume rss content in ASP.NET
Here’s a simple program that can consume and display it in your ASP.NET application
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Xml;
using System.Xml.XPath;
namespace ReadRssContents
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
//txtRssUrl.Text = "http://www.msdn.com/rss.xml";
}
protected void btnFetchRss_Click(object sender, EventArgs e)
{
XPathDocument xpDoc = new XPathDocument(GetRssUrl());
XPathNavigator xpNav = xpDoc.CreateNavigator();
XPathExpression xpExpr = xpNav.Compile("/rss/channel/item");
XPathExpression xpTitle = xpNav.Compile("string(title/text())");
XPathExpression xpLink = xpNav.Compile("string(link/text())");
XPathExpression xpDesc = xpNav.Compile("string(description/text())");
XPathNodeIterator iter = xpNav.Select(xpExpr);
while (iter.MoveNext())
{
string sTitle = (string)iter.Current.Evaluate(xpTitle);
string sLink = (string)iter.Current.Evaluate(xpLink);
string sDescription = (string)iter.Current.Evaluate(xpDesc);
TableRow tRow = new TableRow();
TableCell tCell = new TableCell();
HyperLink hLink = new HyperLink();
hLink.Text = sTitle;
hLink.NavigateUrl = sLink;
tCell.Controls.Add(hLink);
Label lblDescription = new Label();
lblDescription.Text = sDescription;
tCell.Controls.Add(lblDescription);
tRow.Cells.Add(tCell);
tblRssResults.Rows.Add(tRow);
}
}
private string GetRssUrl()
{
return txtRssUrl.Text;
}
}
}
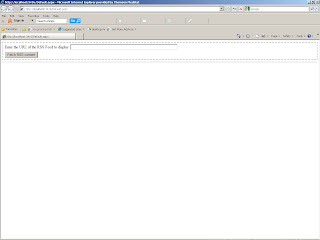
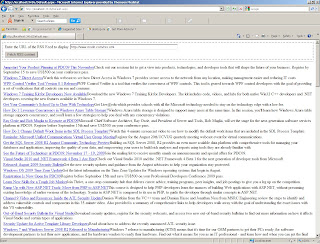